Qualtrics (Part 1)
HTML and CSS in Qualtrics
Example: Improve survey response rate by hiding extra content
HTML:
What is your
<span
class='moreInfo'
title='Primary language is the language a person learns from birth or within their critical period of language development. It is the language spoken in the person’s home or community during their early years and typically becomes the primary means of communication and cultural identification.'>
primary
</span>
language?
Edit CSS via Look & Feel → Style → Custom CSS
.moreInfo {
border-bottom: 2px solid black;
cursor: pointer;
}
.moreInfo::after {
content: "*"; /* Add an asterisk symbol */
font-size: 0.8em; /* Make it slightly smaller */
margin-left: 5px; /* Add some space between text and asterisk */
vertical-align: super; /* Align it slightly higher */
}
Learn more: Qualtrics Docs > Add Custom CSS
Link to your jsPsych experiment from a Qualtrics survey
Create a link to your experiment from Qualtrics - E.g.:
<a target='_blank' href='https://susanbuck.github.io/psy1903/projects/lexical-decision?qualtricsId=${e://Field/ResponseID}
'>Please visit this link and follow the instructions you see there...</a>
Observe:
- The attribute
_target='_blank'
is used to force the link to open in a new tab so the respondent does not exit the survey. - The query string
?qualtricsId=${e://Field/ResponseID}
is added to attach a Qualtrics response ID to the link - The code
${e://Field/ResponseID}
is syntax for Qualtrics Piped Text which you can read about here...
To capture the response ID in your jsPsych experiment, add the following code at the top of your experiment JavaScript file:
// Retrieve the query string from the URL
let queryString = new URLSearchParams(window.location.search);
// Extract the value for qualtricsId from the query string
let qualtricsId = queryString.get('qualtricsId');
// Persist the value for qualtricsId to your experiment data
jsPsych.data.addProperties({ qualtricsId: qualtricsId });
A downside of this approach is the respondent might fail to return to the survey to complete it. A way to avoid this to put the link to the experiment as part of your survey end message.
Alternatively, you could embed your experiment directly in the Qualtrics survey (see below).
Learn more: Passing information from a Survey
Embed your jsPsych experiment in a Qualtrics survey
Using a HTML iframe element, you can embed your jsPsych experiment directly in a question within your Qualtrics survey - E.g.:
<iframe
src='https://susanbuck.github.io/psy1903/projects/lexical-decision/?qualtricsId=${e://Field/ResponseID}'
id='experimentEmbed'>
</iframe>
CSS to style the iframe:
#experimentEmbed {
width: 100%;
height: 500px;
}
Observe how just like with the link, we’re attaching a Qualtrics ID that can be attached to the experiment results (see code from the above example) so we can make the connection between the survey and the experiment.
Link to Qualtrics survey from your jsPsych experiment
Instead of linking from Qualtrics to your experiment, you could go the other direction, having participants start on your experiment and then link to Qualtrics.
For this, you will want to pass info into your Qualtrics survey so you can make a connection between the survey and the experiment.
To do this, start by creating an Embedded Data element in Qualtrics:
Survey Flow → Add a New Element Here → Embedded Data
We’ll name the new embedded data experimentParticipantId
, and move it to the top of the Survey Flow.
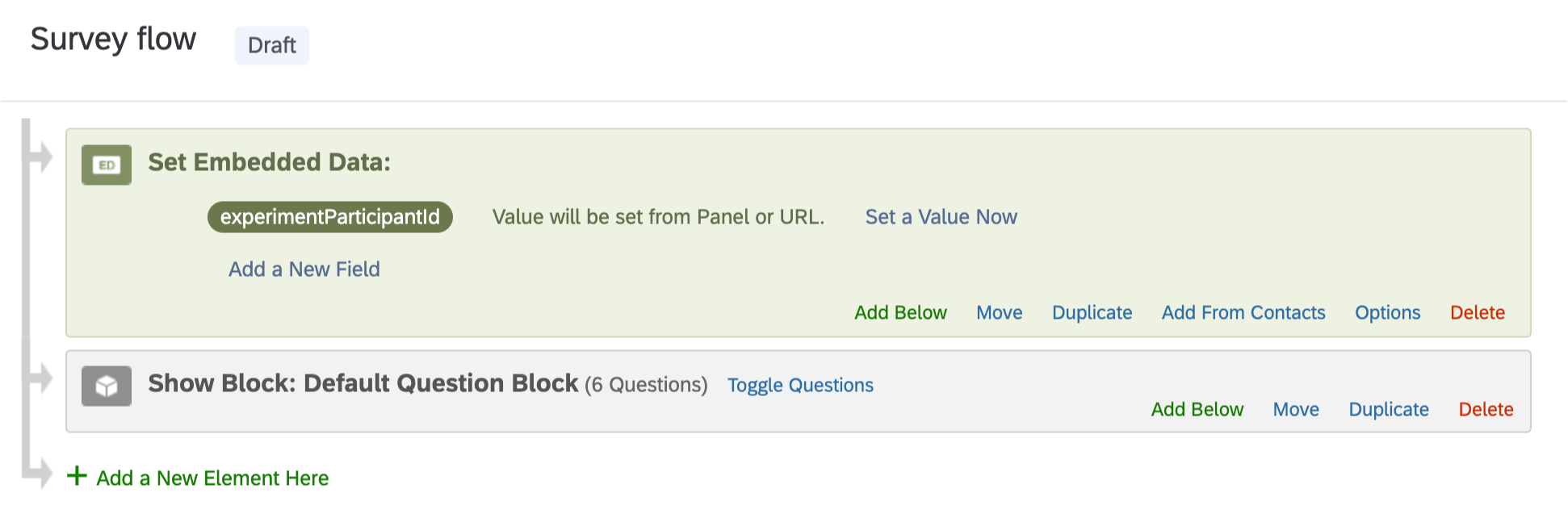
Next, we’ll update our experiment code to link to the Qualtrics survey, attaching a experimentParticipantId
value as part of the query string.
In your experiment JavaScript, define participantId
as a global variable at the top of your file:
let participantId = getCurrentTimestamp();
In your results trial, remove the line defining participantId (since it is now defined globally).
Finally, in your debrief trial, link to your survey adding a experimentParticipantId
query string with the participantId
:
let debriefTrial = {
type: jsPsychHtmlKeyboardResponse,
stimulus: function (data) {
let linkToQualtricsSurvey = `https://harvard.az1.qualtrics.com/jfe/form/SV_ekV0pVH9xqAoOb4?experimentParticipantId=${participantId}`
return `
<h1>Thank you!</h1>
<p>
To complete your response,
please follow <a href='${linkToQualtricsSurvey}'>this link</a>
and complete the survey you see there.
</p>
`},
choices: ['NO KEYS']
}
timeline.push(debriefTrial);
Learn more: Passing information into a Survey