Programmer’s Mindset
We want to begin this course by talking about the process of learning to code. We find it’s useful to develop a certain mindset when programming, because for many people, it’s an entirely new way of communicating that may be frustrating at first.
And so, the following thoughts might be useful as you begin this journey...
Coding takes practice
Coding is challenging, and it takes time to get good at it. Think about some skill you’ve learned in life, like how to play a musical instrument, learn a new language, run 5 miles, etc.
Were you good at these skills after reading a book, taking a single course, or going for a training run?
Chances are the answer is no, and you had to work at that skill, making mistakes and learning as you went. It was probably frustrating at first, and maybe you thought you weren’t cut out for it.
But if you stuck with it, you got better, little by little. Eventually the fun of producing something interesting outweighed the frustrations of making mistakes.
Coding is no different from any of these skills, so remind yourself about that as you’re starting out. If you feel like everything you’re trying is failing, it's not because you’re not fit to be a coder, it’s just because it takes practice.
Failing is a normal part of coding
Take a moment to think about the process of stone carving. With each maneuver, the artist chips away at material, working towards some end shape.
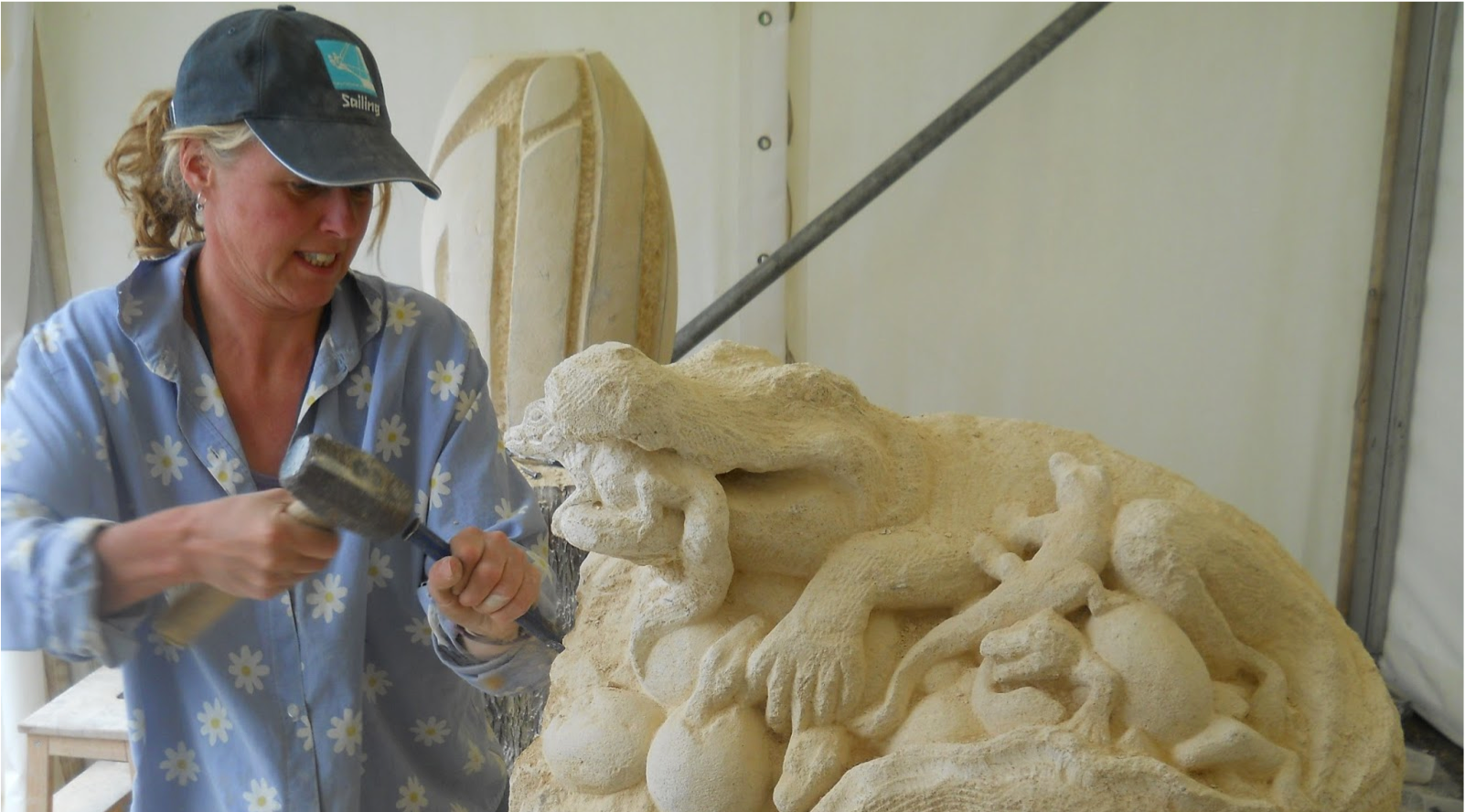
There are some ways to work around errors in this subtractive process, but generally speaking, each move should be purposeful because there’s no “undo” option. It stresses us out just thinking about it!
Fortunately, programming is more forgiving than stone carving. The code you write will rarely work the first time, and that’s part of the process.
If you watch an experienced programmer at work, you’ll see them write a small amount of code, test it out, and discover it fails. Without any hesitation or disappointment, they’ll go right back to the code, change something, then test it again. On and on the cycle continues, until they’ve worked out the kinks, and then they move on to the next part of the task.
Of course, sometimes the cycle goes on for too long and even experienced programmers will get frustrated. When this happens, though, it’s typically a sign that we need to take a break, talk about the problem out loud with a peer, or even sleep on it.
So keep this in mind as you start writing code: It’s perfectly normal for it to fail, and fail, and even fail some more. Try not to think of failing code as broken, but rather, unfinished.
Think like a computer
A common mistake that new coders make is assuming too much about a computer’s ability to fill in the gaps in what we’re asking it to do. To demonstrate what we mean, consider this short story:
A man asks his partner (who’s a programmer) to go to the grocery store. He tells her to get a gallon of milk, and, if they have eggs, to get a dozen.
When she gets back, she has 12 gallons of milk. When he asks why, she says:
“They had eggs.”
See what happened there? The man was relying on assumptions based on how we, as humans, communicate. Our thought processes have all these shortcuts to make us more efficient, and we rely on other humans to be able to parse this information and come to logical conclusions about what we mean.
You’ll find that when giving instructions to a computer (i.e. coding), the computer will be a lot less forgiving of the shortcuts you're accustomed to. Here’s a programming related example...
Let’s say you’re filtering some data and only want values between 50 and 100.
In code, you’d communicate this True/False expression as...
if value > 50 and value < 100...
But as humans, we’d typically shorten this expression to...
if value > 50 and < 100...
Do you see how the first example is very explicit in what we’re comparing, while the second example makes assumptions that both 50 and 100 should be compared to price?
When you first start out, you’ll fall into these little gotchas frequently. When that happens, take a step back and examine what you’re asking the computer to do, and whether you’ve provided all the information it needs.
Our goals
One final note as you prepare to delve into the technical material...
The goal of this course is not to be a comprehensive guide to JavaScript or R; there are already many great resources for that, and it’s not realistic to expect to master two languages in the span of a semester.
Our goal, then, is to provide a springboard into the world of coding and programmatic thinking. We will introduce you to some, but not all, fundamental programming concepts, and tackle exercises that show these concepts at work.
More than the specific concepts though, we also want to plant the following seeds:
- Strategizing: How to attack problems, breaking them down into manageable parts
- Learning: How to read documentation and become more fluent in learning new technical concepts
- Troubleshooting: How to effectively search for solutions on Google, programming forums, via AI, etc.
- Debugging: How to get feedback from your code when it’s not doing what you’re expecting