jsPsych plugin: survey-html-form
Primarily, we’ve been using jsPsych’s html-keyboard-response plugin.
In the Week 5 task set, we will also use the survey-html-form plugin, so this note set provides guidance on how to use that plugin.
From the jsPsych docs, we learn that the survey-html-form plugin lets us display form inputs and collect data from these inputs.
For a simple example, we will utilize this plugin to ask the participant to enter their age.
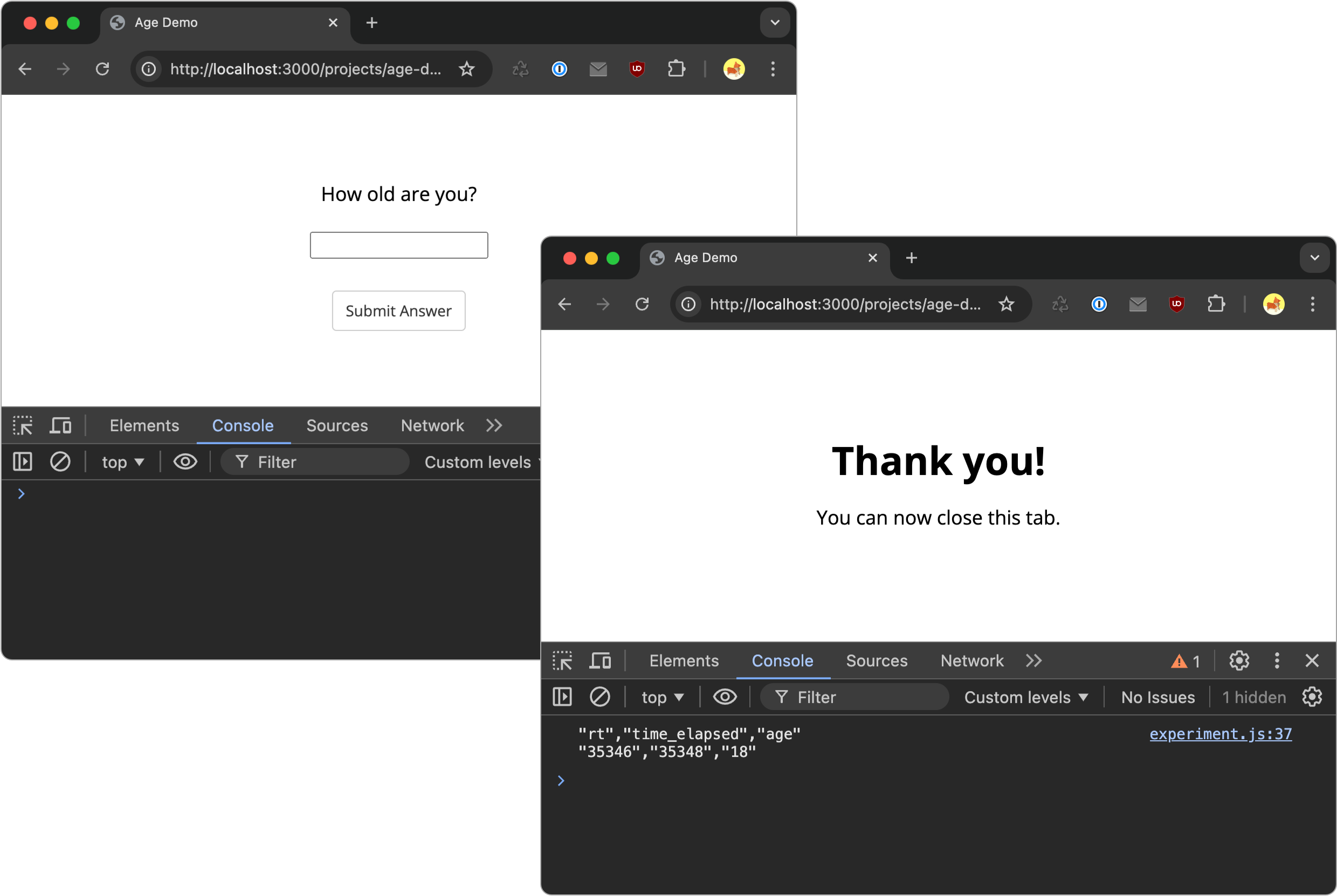
HTML
<!DOCTYPE html>
<html>
<head>
<title>Age Demo</title>
<link href=data:, rel=icon>
<link href='https://unpkg.com/jspsych/css/jspsych.css' rel='stylesheet' type='text/css'>
<script src='https://unpkg.com/jspsych'></script>
<script src='https://unpkg.com/@jspsych/plugin-html-keyboard-response'></script>
<!-- ⭐ NEW ⭐ -->
<script src='https://unpkg.com/@jspsych/plugin-survey-html-form'></script>
<script src='experiment.js'></script>
</head>
<body></body>
</html>
JavaScript
let jsPsych = initJsPsych();
let timeline = [];
let ageTrial = {
type: jsPsychSurveyHtmlForm,
preamble: '<p>How old are you?</p>',
html: `<p><input type='text' name='age' id='age'></p>`,
autofocus: 'age', // id of the field we want to auto-focus on when the trial starts
button_label: 'Submit Answer',
data: {
collect: true,
},
on_finish: function (data) {
data.age = data.response.age;
}
}
timeline.push(ageTrial);
// Debrief
let debriefTrial = {
type: jsPsychHtmlKeyboardResponse,
stimulus: `
<h1>Thank you!</h1>
<p>You can now close this tab.</p>
`,
choices: ['NO KEYS'],
on_start: function () {
let data = jsPsych.data
.get()
.filter({ collect: true })
.ignore(['response', 'stimulus', 'trial_type', 'trial_index', 'plugin_version', 'collect'])
.csv();
console.log(data);
}
}
timeline.push(debriefTrial);
jsPsych.run(timeline);