Page Input and Output
So far, we’ve been using some ”quick and dirty” ways to collect input and produce output in the browser using methods like prompt
, console.log
, and alert
.
Building on this, let’s expand our abilities to collect input and report results directly to the page.
To set up an example, create a new directory at psy1903/docs/projects/input-output
with the following two files:
index.html
app.js
Within index.html
place this code:
<!doctype html>
<html lang='en'>
<head>
<title>Input/Output Practice</title>
<link href=data:, rel=icon>
<script src='app.js' defer></script>
</head>
<body>
<h1>Input/Output Practice</h1>
<form>
What is your name?
<input type='text' name='response'>
<button type='submit'>Submit</button>
</form>
<p id='results'></p>
</body>
</html>
Within app.js
, place this code:
// Create variables to store references to elements on the page
let form = document.getElementsByTagName('form')[0];
let results = document.getElementById('results');
// Listen for the form to be submitted
form.addEventListener('submit', function (event) {
// Prevent the default form submission b
event.preventDefault();
// Collect the response
let response = form.elements['response'].value;
// Report the results
results.innerHTML = 'Hello ' + response + '!';
});
When you load the above setup in your browser, you should be able to enter your name where prompted, and see a welcome message upon submitting the form:
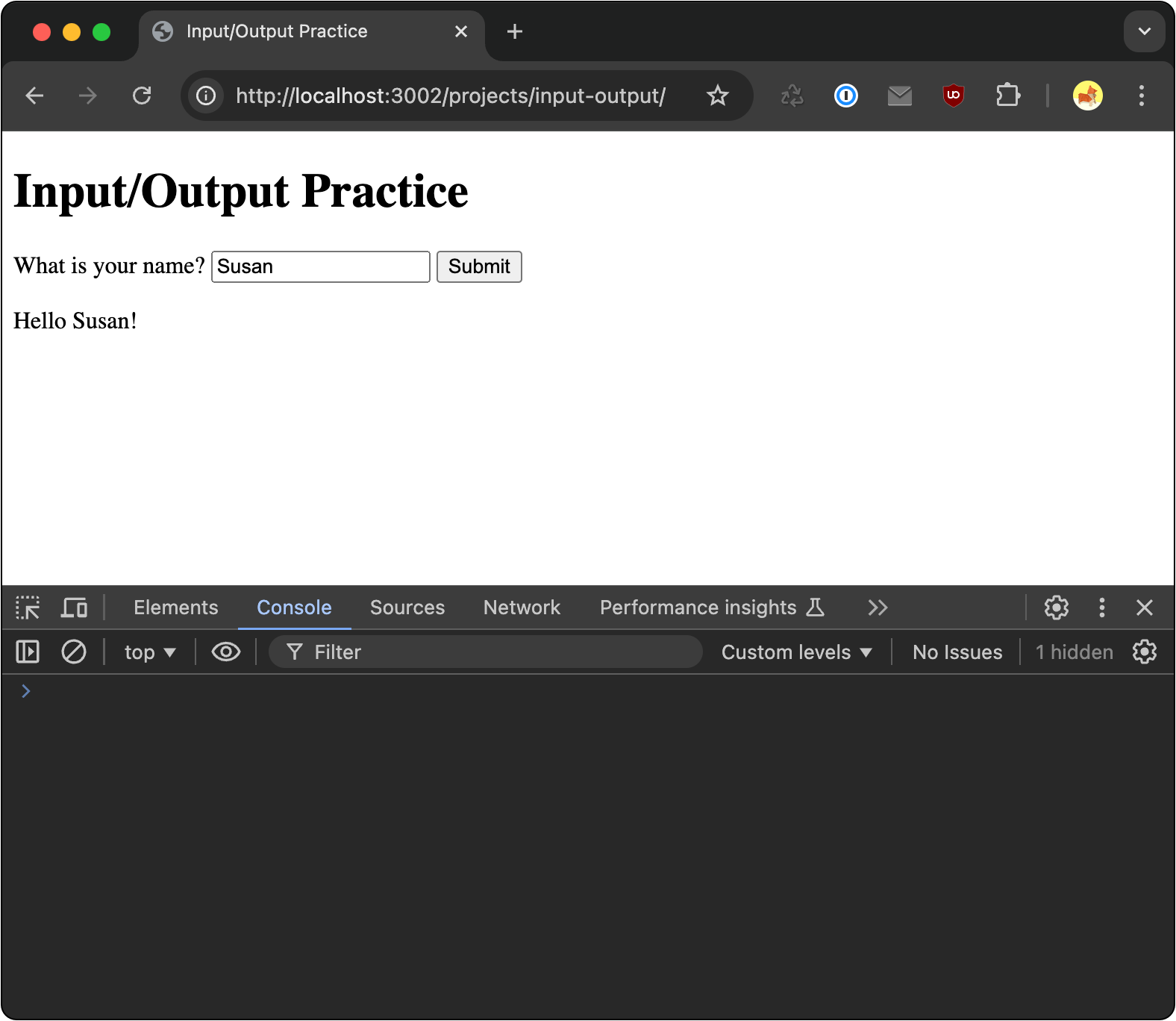
Observe how even though we’re now outputting content to the page, we are still keeping the web inspector open. This way, if our code has any errors we’ll be able to see details about that error in the console.
Let’s breakdown what the above code is doing...
Selecting elements in the document
An essential task for JavaScript is to manipulate content on the page. This is accomplished using JavaScript’s document interface which gives you access to the entire Document Object Model (DOM) of the HTML page.
The DOM represents every HTML element on the page, and it can be visualized as a tree. Example:
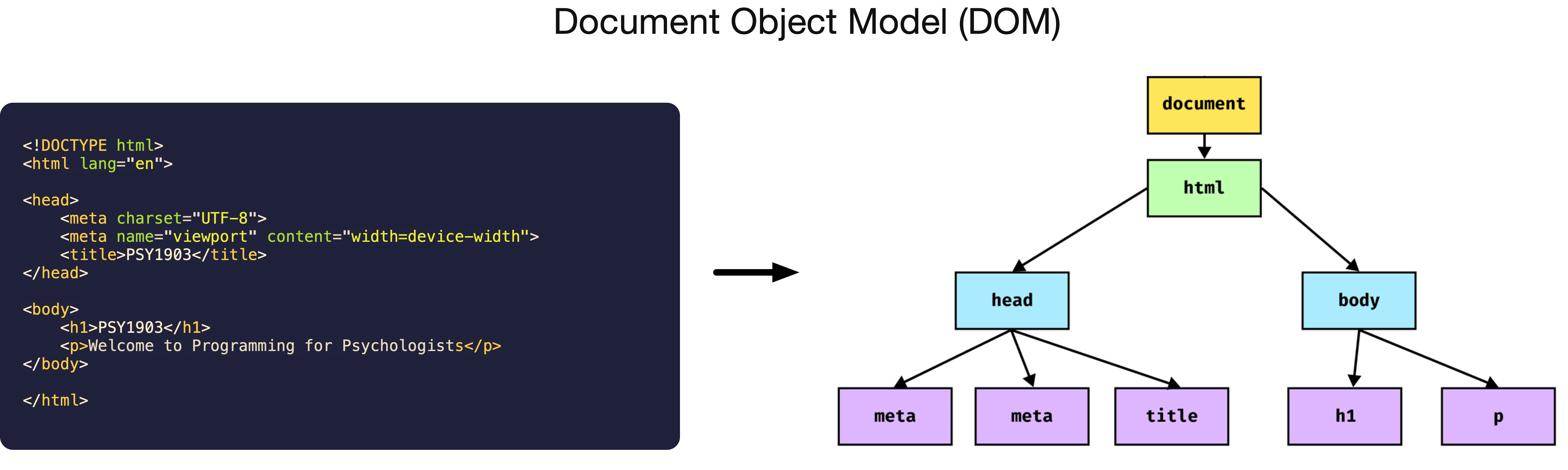
JavaScript’s document interface has different methods for targeting elements on the page.
For example, in the above JavaScript code we used the document method getElementsByTagName to “select” the form on the page and store a reference to it in the variable named form
:
let form = document.getElementsByTagName('form')[0];
Corresponding HTML element:
<form>
What is your name?
<input type='text' name='response'>
<button type='submit'>Submit</button>
</form>
We also selected the results paragraph using the getElementById method:
let results = document.getElementById('results');
Corresponding HTML element:
<p id='results'></p>
Once we have a reference to an element on a page, we can work with that element in a variety of ways.
For example, with the form element, we attached a "submit" event listener (via the method addEventListener), which allowed us to execute some code when the form was submitted:
// Listen for the form to be submitted
form.addEventListener('submit', function (event) {
// Prevent the default form submission
event.preventDefault();
// Collect the response from the text input
let response = form.elements['response'].value;
// Report the results
results.innerHTML = 'Your response:' + response;
});
Within the event listener we were able to access the value from the response input, and update the results paragraph with that response:
// Collect the response from the text input
let response = form.elements['response'].value;
// Report the results
results.innerHTML = 'Your response:' + response;
Summary
This practice of using JavaScript to select elements on the page and manipulate them in some way is the keystone of pretty much every interactive website you encounter. As we dig deeper, we will encounter different approaches to selecting elements and learn more about the different actions we can perform with those elements.