Miscellaneous
The following is a list of miscellaneous jsPsych techniques to have on your radar.
Conditionally execute trials
Example: Only certain participants, chosen at random, will be shown a particular trial
let primeTrial = {
type: jsPsychHtmlKeyboardResponse,
stimulus: `
<p>You were randomly chosen to see this trial.</p>
<p>Press the <span class='key'>SPACE</span> key to continue.</p>
`,
choices: [' '],
data: {
collect: true,
trialType: 'prime',
},
on_load: function () {
if (getRandomNumber(0, 1) == 0) {
jsPsych.data.addProperties({ sawPrime: false });
jsPsych.finishTrial();
} else {
jsPsych.data.addProperties({ sawPrime: true });
}
}
}
timeline.push(primeTrial);
Example: Only display a feedback trial if the participant answered the previous trial incorrectly
let feedbackTrial = {
type: jsPsychHtmlKeyboardResponse,
stimulus: `<h1>Incorrect</h1>`,
trial_duration: 1000,
choices: ['NO KEY'],
on_load: function () {
let lastTrialData = jsPsych.data.getLastTrialData().values()[0];
if (lastTrialData.correct) {
// Force skip this feedback trial if they got the previous trial correct
jsPsych.finishTrial();
}
},
}
timeline.push(feedbackTrial);
Abort experiment
Example: Abort the experiment if participant is a minor
let ageCheckTrial = {
type: jsPsychSurveyHtmlForm,
html: `
<h1>Welcome!</h1>
Please enter your age to continue: <input type='text' name='age' id='age'>
`,
autofocus: 'age',
on_finish: function (data) {
if (data.response.age < 18) {
jsPsych.abortExperiment('You must be 18 years or older to complete this experiment.');
}
}
}
timeline.push(ageCheckTrial);
Progress bar
Ref: jsPsych Automatic Progress Bar
let jsPsych = initJsPsych({
show_progress_bar: true
});
Update debrief trial, adding an on_start callback that sets the progress bar to 100% (1):
on_start: function () {
jsPsych.progressBar.progress = 1;
}
Full screen experiments
Ref: jsPsych Full Screen Experiments; plugin fullscreen
Add the full screen plugin to your HTML:
<script src='https://unpkg.com/@jspsych/plugin-fullscreen'></script>
Add a trial to initiate full screen:
let enterFullScreenTrial = {
type: jsPsychFullscreen,
fullscreen_mode: true
};
timeline.push(enterFullScreenTrial);
At the end, before your debrief trial, add a trial to close full screen:
let exitFullScreenTrial = {
type: jsPsychFullscreen,
fullscreen_mode: false
};
timeline.push(exitFullScreenTrial);
Safari does not support keyboard input when the browser is in fullscreen mode. Therefore, the function will not launch fullscreen mode on Safari. The experiment will ignore any trials using the fullscreen plugin in Safari.
Virtual Chin Rest
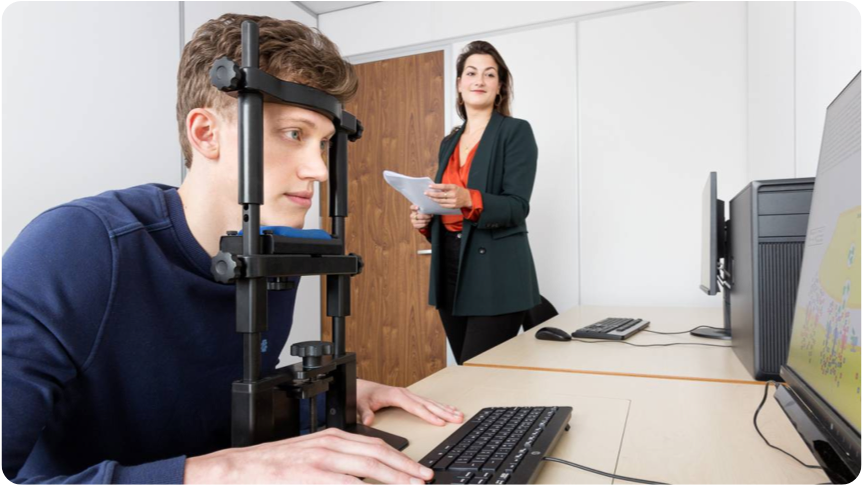
The jsPsych virtual chinrest is used to estimate the physical distance between a participant’s eyes and their computer screen. This is important in psychological and vision experiments where precise control over visual stimuli (such as their size or position) is critical for ensuring that results are valid.
Since online experiments cannot control the actual physical setup of participants, the virtual chinrest provides a way to estimate screen distance and adjust visual stimuli accordingly.
Learn more: https://www.jspsych.org/latest/plugins/virtual-chinrest/