Conditions
To set up the core of our experiment, let’s start by defining an array of conditions, which for the purposes of our lexical decision task, will look like this:
// Create an array of conditions
let conditions = [
{ characters: 'cat', isWord: true },
{ characters: 'pin', isWord: true },
{ characters: 'jgb', isWord: false },
{ characters: 'mub', isWord: false },
];
// Shuffle the conditions
conditions = jsPsych.randomization.repeat(conditions, 1);
To begin, we start with a small subset of conditions so that testing our experiment can be done quickly. Later, we can expand on this.
Observe how jsPsych’s randomization.repeat method is used to shuffle our conditions so they’re presented in a random order.
Loop conditions
Using our array of conditions, let’s define a loop that will initialize a new html-keyboard-response plugin trial for each condition:
for (let condition of conditions) {
let conditionTrial = {
type: jsPsychHtmlKeyboardResponse,
stimulus: `<h1>${condition.characters}</h1>`,
// Listen for either the f or j key to proceed
choices: ['f', 'j'],
};
timeline.push(conditionTrial);
}
Run your experiment in the browser to test your work. You should see the welcome trial, followed by each of your conditions. As instructed, press the f or j key to proceed through the conditions. The experiment still ends on a blank screen, so let’s add a debrief trial.
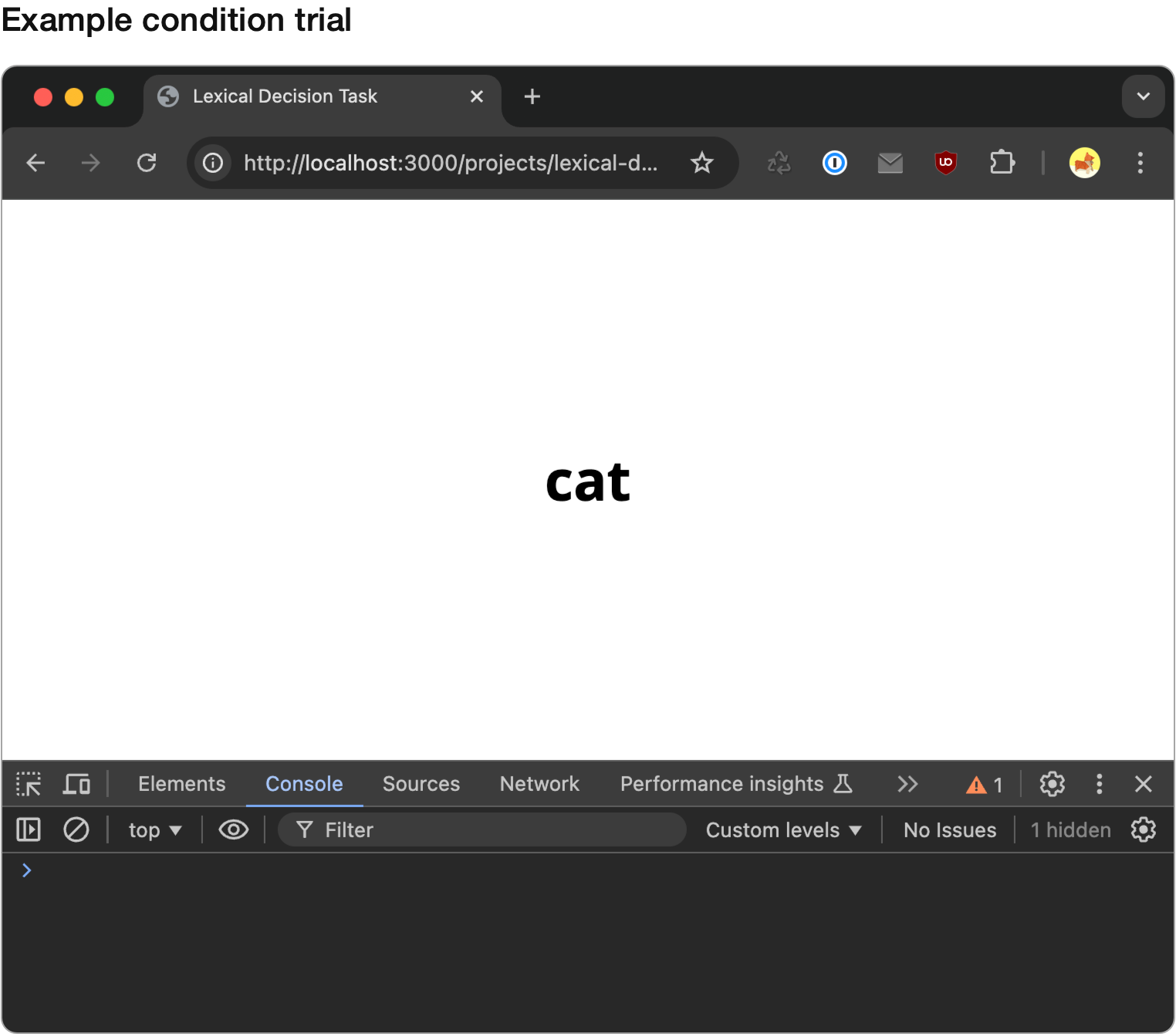
Debrief trial
Here’s code for a debrief trial:
let debriefTrial = {
type: jsPsychHtmlKeyboardResponse,
stimulus: `
<h1>Thank you for participating!</h1>
<p>You can close this tab.</p>
`,
choices: ['NO KEYS'],
};
timeline.push(debriefTrial);
Observe how for the choices
parameter, we passed a special keyword 'NO KEYS'
indicating that nothing will happen on this screen when keys are pressed.
This is what the debrief trial looks like:
Next...
Our rudimentary experiment is now complete, so let’s now turn our attention to accessing the results of the experiment...
Code so far
// Initialize the jsPsych library
let jsPsych = initJsPsych();
// Create timeline
let timeline = [];
let welcomeTrial = {
// Indicate the plugin type we’re using
type: jsPsychHtmlKeyboardResponse,
// What stimulus to display on the screen
stimulus: `
<h1>Welcome to the Lexical Decision Task!</h1>
<p>You are about to see a series of characters.</p>
<p>If the characters make up a word, press the F key.</p>
<p>If the characters do not make up a word, press the J key.</p>
<p>Press SPACE to begin.</p>
`,
// Listen for the SPACE key to be pressed to proceed
choices: [' '],
};
timeline.push(welcomeTrial);
// Create an array of conditions
let conditions = [
{ characters: 'cat', isWord: true },
{ characters: 'pin', isWord: true },
{ characters: 'jgb', isWord: false },
{ characters: 'mub', isWord: false },
];
// Shuffle the conditions
conditions = jsPsych.randomization.repeat(conditions, 1);
for (let condition of conditions) {
let conditionTrial = {
type: jsPsychHtmlKeyboardResponse,
stimulus: `<h1>${condition.characters}</h1>`,
// Listen for either the f or j key to proceed
choices: ['f', 'j'],
};
timeline.push(conditionTrial);
}
let debriefTrial = {
type: jsPsychHtmlKeyboardResponse,
stimulus: `
<h1>Thank you for participating!</h1>
<p>You can close this tab.</p>
`,
choices: ['NO KEYS'],
};
timeline.push(debriefTrial);
jsPsych.run(timeline);