Save Results
In the Results note set we learned how to access the results from our experiment and output them to the web inspector console.
This was useful for us to examine/make sure we were collecting the correct data, but of course we will need a way to save these results from each participant so that the data can then be studied and analyzed.
To accomplish this, we will have two modes for saving data:
- In our “development environment” results will be saved as .csv text files to our computer via our local web server.
- In our “production environment” where we’ll share our experiment with real-world participants, the .csv text files will be saved to OSF.io (Open Science Framework), an open-source platform designed to facilitate open and reproducible research.
Setup
Saving our results to text files will require more code than just outputting the results to the console, so let’s re-organize our experiment so the “save results” code is in its own trial rather than embedded in the debrief trial. This re-organization will also allow us to have a “Please wait while we save your results...” message appear while the save process executes.
Before the debriefTrial
, add a new resultsTrial
with the following code:
let resultsTrial = {
type: jsPsychHtmlKeyboardResponse,
choices: ['NO KEYS'],
async: false,
stimulus: `
<h1>Please wait...</h1>
<p>We are saving the results of your inputs.</p>
`,
on_start: function () {
// ⭐ Update the following three values as appropriate ⭐
let prefix = 'lexical-decision';
let dataPipeExperimentId = 'your-experiment-id-here';
let forceOSFSave = false;
// Filter and retrieve results as CSV data
let results = jsPsych.data
.get()
.filter({ collect: true })
.ignore(['stimulus', 'trial_type', 'plugin_version', 'collect'])
.csv();
// Generate a participant ID based on the current timestamp
let participantId = new Date().toISOString().replace(/T/, '-').replace(/\..+/, '').replace(/:/g, '-');
// Dynamically determine if the experiment is currently running locally or on production
let isLocalHost = window.location.href.includes('localhost');
let destination = '/save';
if (!isLocalHost || forceOSFSave) {
destination = 'https://pipe.jspsych.org/api/data/';
}
// Send the results to our saving end point
fetch(destination, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
Accept: '*/*',
},
body: JSON.stringify({
experimentID: dataPipeExperimentId,
filename: prefix + '-' + participantId + '.csv',
data: results,
}),
}).then(data => {
console.log(data);
jsPsych.finishTrial();
})
}
}
timeline.push(resultsTrial);
There’s a lot of new code in this trial which will be explained in more detail in the video that accompanies this note set, but the following is a summary of key points:
- In the
on_start
method, the saving happens. - The plugin parameter
async
is set tofalse
. This means the code that communicates with the server (either our local server or OSF) must complete before the trial will complete. This ensures our data is saved before the experiment continues. - Update the variable
prefix
with the name of your experiment (in this example, it’s set toldt
(short for lexical decision)). This prefix will be used as part of your results filenames. - When this code is run on our development environments via the localhost URL, it will default to piping the results to the
/save
route on our web server, which is pre-programmed to save the results to a file within our project’s/data
directory. - To force data to be saved to OSF.io even when running locally, toggle the variable forceOSFSave to true.
- In order for the saving to OSF.io to work, the same setup steps will need to be completed. We’ll cover details for that in Week 8.
Test local saving
With the above code in place, run through your experiment and then confirm results are being written to psy1903/data
.
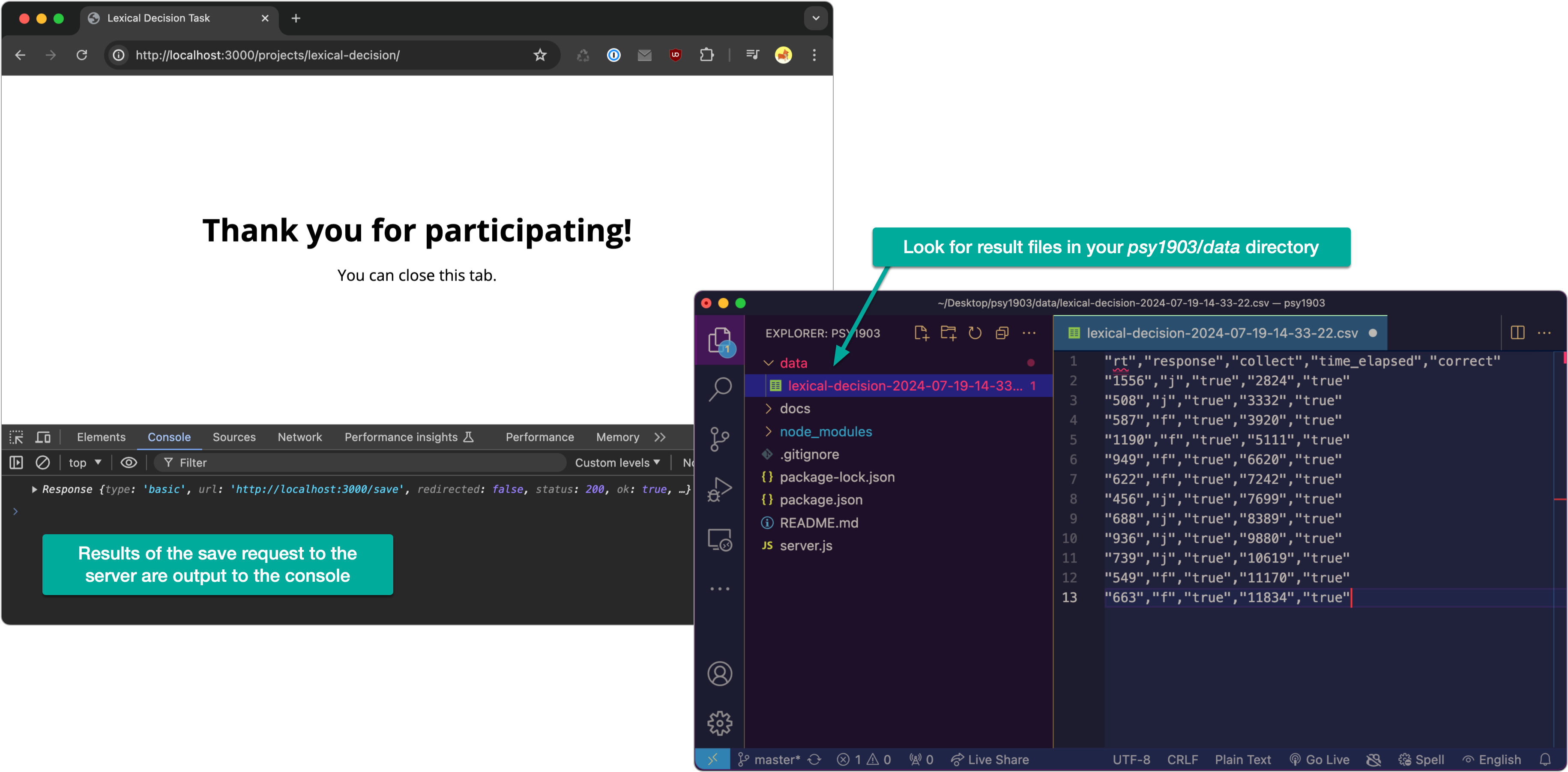
When developing/testing experiments, this is the method you should use for collecting results as it’s quicker and uses less resources then sending the results to OSF.io (to be covered in Week 8).